[TOC]
sparse
kron
1 | from scipy import sparse |
$A \times B$ 如果A是一个m×n的矩阵,而B是一个p×q的矩阵,克罗内克积则是一个mp×nq的分块矩阵
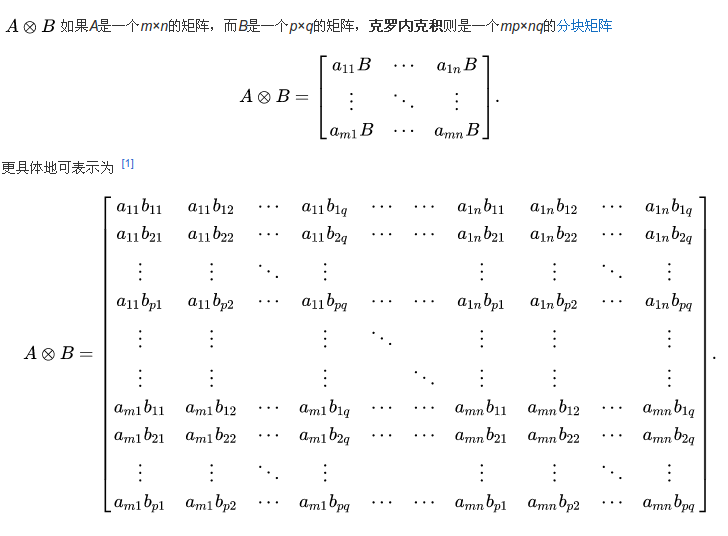
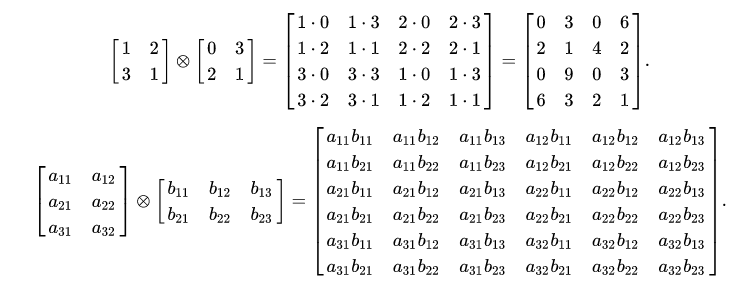
csr_matrix
1 | from scipy.sparse import * |
lil_matrix
1 | 100).reshape(10, 10) a = np.arange( |
安装
1 | sudo apt-get install python-scipy libsuitesparse-dev |
cholesky